Python if
statement#
In control statements, The if
statement is the simplest form. It takes a condition and evaluates to either True
or False
.
If the condition is True
, then the True
block of code will be executed, and if the condition is False
, then the block of code is skipped, and The controller moves to the next line.
Syntax:#
if(condition):
statement 1
statement 2
statement n
An
if
statement should be preceded by the keywordif
and ended with a colon:
. The condition of an if statement can be written with or without round brackets()
.In Python, the body of the
if
statement is indicated by the indentation. The body starts with an indentation and the first unindented line marks the end.Python interprets non-zero values as
True
.None
and0
are interpreted asFalse
.
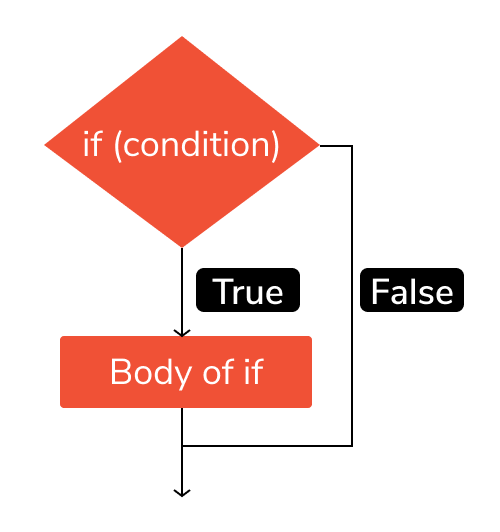
Example: of if
statement:
>>> grade = 70
>>> if grade >= 65:
>>> print("Passing grade")
Passing grade
With this code, we have the variable grade and are giving it the integer value of 70. We are then using the if
statement to evaluate whether or not the variable grade is greater than or equal >=
to 65. If it does meet this condition, we are telling the program to print out the string Passing grade.
Again, if we change the grade to 50 or < 65 number, we will receive no output.
# Example 1:
grade = 70
if grade >= 65:
print("Passing grade")
Passing grade
# Example 2: If the number is positive, we print an appropriate message
num = 3
if (num > 0): # if condition is TRUE: enter the body of if
print(num, "is a positive number.")
print("This is always printed.")
num = -1
if num > 0: # if condition is FALSE: do not enter the body of if
print(num, "is a negative number.")
print("This is also always printed.")
3 is a positive number.
This is always printed.
This is also always printed.
Explanation:
In the above example, num > 0
is the test expression.
The body of if
is executed only if this evaluates to True
.
When the variable num
is equal to 3, test expression is true and statements inside the body of if
are executed.
If the variable num
is equal to -1, test expression is false and statements inside the body of if
are skipped.
The print() statement falls outside of the if
block (unindented). Hence, it is executed regardless of the test expression.
# Example 3: Calculate the square of a number if it greater than 6
number = 9
if number > 6:
# Calculate square
print(number * number)
print('Next lines of code')
81
Next lines of code
# Example 4: Check if num1 is less than num2
num1, num2 = 6, 9
if(num1 < num2):
print("num1 is less than num2")
num1 is less than num2
x = 12
if x > 10:
print("Hello")
Hello
if 5 != 3 * 6:
print ("Hooray!")
Hooray!
if 5 == 15/3:
print ("Horray!")
Horray!
Shortcut for if
statement (Short-hand if
or one-line if
)#
If you have only one statement to execute, then you can put it on the same line as the if
statement. Let’s try doing this for the above example.
num1, num2 = 5, 6
if(num1 < num2): print("num1 is less than num2")
num1 is less than num2
The Difference…#
Now, try to execute the below-given code and observe the difference.
#program to check if num1 is less than num 2
num1, num2 = 6, 5
if (num1 < num2):
print("num1 is less than num2")
Explanation:
Did your Python IDE print anything? No, it will not.
In the above-given code, the value 6 is greater than 5, this means the condition is False. We have instructed the interpreter to print only when the condition is True and hence it in this case, nothing gets printed. However, you can also instruct the interpreter what to do when the condition is False and this can be done using else statement.
if 5 == 18/3:
print ("Hooray!")