Control Statements in Python#
Loops in Python are used to iterate repeatedly over a block of code. But at times, you might want to shift the control once a particular condition is satisfied. This is where control statements in Python come into the picture.
Control statements in python are used to control the flow of execution of the program based on the specified conditions. Python supports 3 types of control statements:
Statement |
Description |
|
---|---|---|
1 |
|
Terminate the current loop. Use the |
2 |
|
Skip the current iteration of a loop and move to the next iteration |
3 |
|
Do nothing. Ignore the condition in which it occurred and proceed to run the program as usual |
Loops iterate over a block of code until the test expression is False
, but sometimes we wish to terminate the current iteration or even the whole loop without checking test expression.
The break
and continue
statements are part of a control flow statements that helps you to understand the basics of Python.
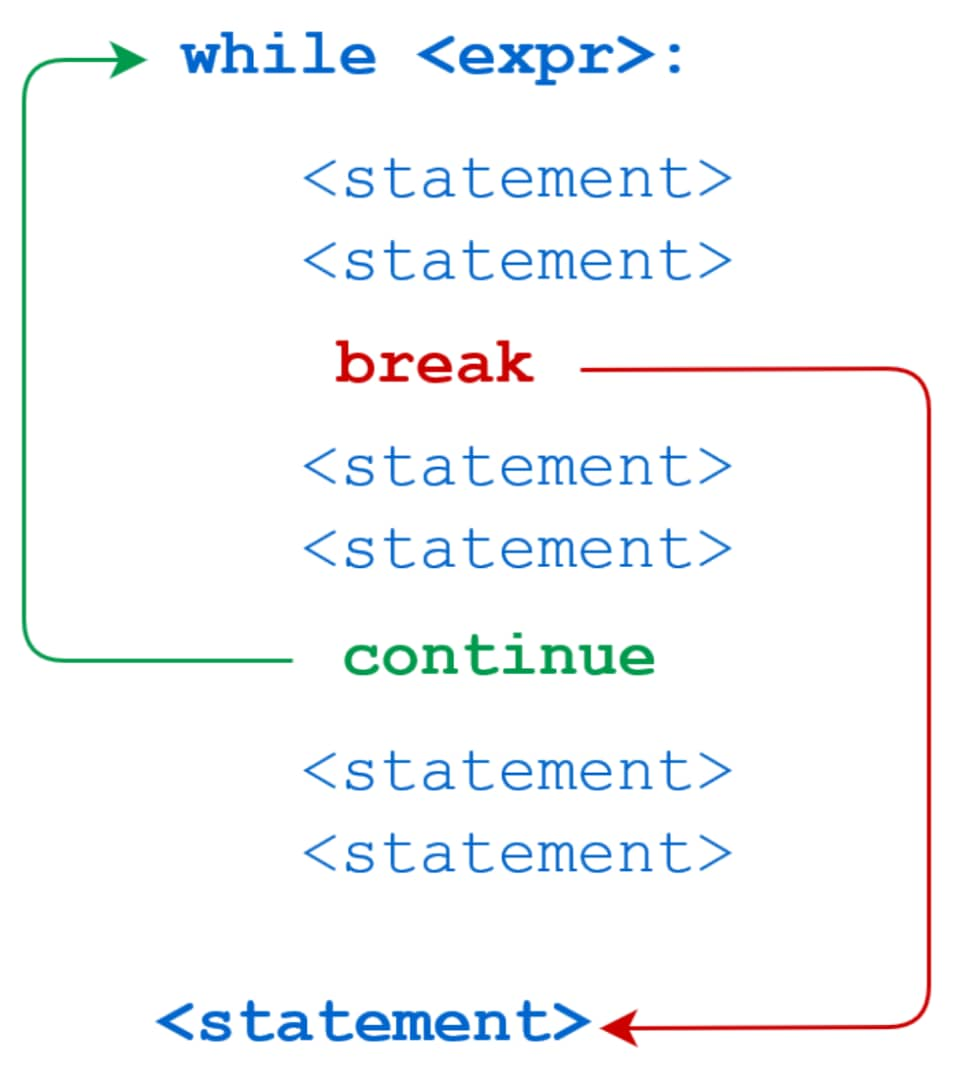
Python break
statement#
In Python, the break
statement provides you with the opportunity to exit out of a loop when an external condition is triggered, and the program control transfer to the next statement following the loop.
If the break
statement is inside a nested loop (loop inside another loop), the break
statement will terminate the innermost loop.
You’ll put the break
statement within the block of code under your loop statement, usually after a conditional if statement. This statement can only be used in for loop and while loop.
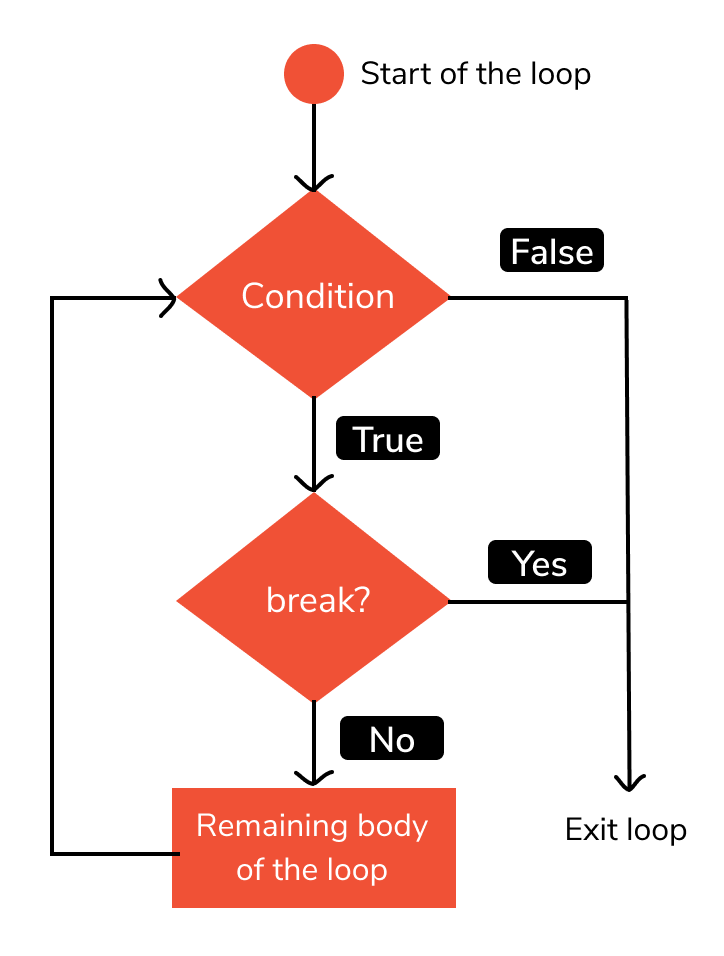
The working of break
statement in for
loop and while
loop is shown below.
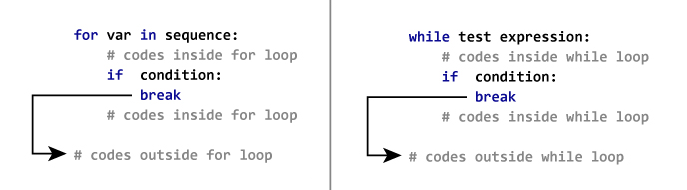
# Example 1:
numbers = [10, 30, 120, 330]
for i in numbers:
if i > 100:
break
print('current number', i)
current number 10
current number 30
# Example 2: Let’s look at an example that uses the break statement in a `for` loop
number = 0
for number in range(10):
number = number + 1
if number == 5:
break # break here
print('Number is ' + str(number))
print('Out of loop')
Number is 1
Number is 2
Number is 3
Number is 4
Out of loop
Explanation:
In this small program, the variable number is initialized at
0
. Then afor
statement constructs the loop as long as the variable number is less than 10.Within the
for
loop, the number increases incrementally by1
with each pass because of the line number = number + 1.Then, there is an
if
statement that presents the condition that if the variable number is equivalent to the integer5
, then the loop will break.Within the loop is also a print() statement that will execute with each iteration of the
for
loop until the loop breaks, since it is after thebreak
statement.To see when we are out of the loop, we have included a final
print()
statement outside of thefor
loop.
This shows that once the integer number is evaluated as equivalent to 5
, the loop breaks, as the program is told to do so with the break
statement.
Nested break
loops#
1. break
Statement in Nested Loop#
To terminate the nested loop, use a break
statement inside the inner loop. For example:
# Example 1:
for i in range(100):
print(i,end="...")
if i>=10:
break
print("completed.")
0...1...2...3...4...5...6...7...8...9...10...completed.
# Example 2: Use of break statement inside the loop
for val in "string":
if val == "i":
break
print(val)
print("The end")
s
t
r
The end
Explanation:
In this program, we iterate through the “string
” sequence. We check if the letter is i
, upon which we break from the loop. Hence, we see in our output that all the letters up till i
gets printed. After that, the loop terminates.
Print the letters in “HELLOWORLD” one by one untill the letter R is reached using break statement in Python.
# Example 3:
for letter in "HELLOWORLD": # letter is a variable which could have been with any name. each iteration the letter is advanced in the string "FACEPREP" and stored in variable letter.
if letter == "R": #check for condition
break # if condition true, then break out of the loop and print the final print statement.
print(letter) # if condition false, then print that letter.
print('untill letter R printed') #once the for loop is terminated, print this.
# Note: observe the indentations carefully
H
E
L
L
O
W
O
untill letter R printed
# Example 4: program to check if letter 'A' is present in the input
a = input ("Enter a word: ")
for i in a:
if (i == 'A'):
print ("A is found")
break
else:
print ("A not found")
# Note: Python is case-sensitive language.
Enter a word: Happy
A not found
# Example 5: bested `while` loop with `break`
name = 'Alan99 White'
size = len(name)
i = 0
# iterate loop till the last character
while i < size:
# break loop if current character is space
if name[i].isspace():
break
# print current character
print(name[i], end=' ')
i = i + 1
A l a n 9 9
# Example 6:
for i in range(1, 11):
print('Multiplication table of', i)
for j in range(1, 11):
# condition to break inner loop
if i > 5 and j > 5:
break
print(i * j, end=' ')
print('')
Multiplication table of 1
1 2 3 4 5 6 7 8 9 10
Multiplication table of 2
2 4 6 8 10 12 14 16 18 20
Multiplication table of 3
3 6 9 12 15 18 21 24 27 30
Multiplication table of 4
4 8 12 16 20 24 28 32 36 40
Multiplication table of 5
5 10 15 20 25 30 35 40 45 50
Multiplication table of 6
6 12 18 24 30
Multiplication table of 7
7 14 21 28 35
Multiplication table of 8
8 16 24 32 40
Multiplication table of 9
9 18 27 36 45
Multiplication table of 10
10 20 30 40 50
Explanation:
We have two loops, the outer loop, and the inner loop. The outer for loop iterates the first 10 numbers using the range() function, and the internal loop prints the multiplication table of each number.
But if the current number of both the outer loop and the inner loop is greater than 5 then terminate the inner loop using the break
statement.
2. break
Statement in Outer Loop#
To terminate the outside loop, use a break
statement inside the outer loop. For example:
# Example 1: Break nested loop
for i in range(1, 11):
# condition to break outer loop
if i > 5:
break
print('Multiplication table of', i)
for j in range(1, 11):
print(i * j, end=' ')
print('')
Multiplication table of 1
1 2 3 4 5 6 7 8 9 10
Multiplication table of 2
2 4 6 8 10 12 14 16 18 20
Multiplication table of 3
3 6 9 12 15 18 21 24 27 30
Multiplication table of 4
4 8 12 16 20 24 28 32 36 40
Multiplication table of 5
5 10 15 20 25 30 35 40 45 50
Explanation:
We have two loops, the outer loop, and the inner loop. The outer loop iterates the first 10 numbers, and the internal loop prints the multiplication table of each number.
But if the current number of the outer loop is greater than 5 then terminate the outer loop using the break
statement.
# Example 2:
numbers = [3, 6, 12, 9]
for i in numbers:
print('Current Number is:', i)
# skip below statement if number is greater than 10
if i > 10:
continue
square = i * i
print('Square of a current number is:', square)
Current Number is: 3
Square of a current number is: 9
Current Number is: 6
Square of a current number is: 36
Current Number is: 12
Current Number is: 9
Square of a current number is: 81
# Example 3: Python Continue Statement Example:
a=0
while a<=5:
a=a+1
if a%2==0:
continue
print (a)
print ("End of Loop")
1
3
5
End of Loop
Explanation:
You can use the continue
statement to avoid deeply nested conditional code, or to optimize a loop by eliminating frequently occurring cases that you would like to reject.
The continue
statement causes a program to skip certain factors that come up within a loop, but then continue through the rest of the loop.
# Example 4: Program to show the use of continue statement inside loops
for val in "string":
if val == "i":
continue
print(val)
print("The end")
s
t
r
n
g
The end
Explanation:
This program is same as the above example except the break
statement has been replaced with continue
.
We continue with the loop, if the string is i
, not executing the rest of the block. Hence, we see in our output that all the letters except i
gets printed.
# Example 5:
for i in range(10):
if i>4:
print("Ignored",i)
continue
# this statement is not reach if i > 4
print("Processed",i)
Processed 0
Processed 1
Processed 2
Processed 3
Processed 4
Ignored 5
Ignored 6
Ignored 7
Ignored 8
Ignored 9
Write a program to skip all “O”s in a given string “HELLOWORLD”
# Example 6:
for letters in "HelloWorld": # for every letter in FACEPREP
if letters == "O": # if letter is E, then skip printing the letter and go to the next iteration with the next alphabet
continue # Remember that loop is not terminated like break statement.
print(letters)
print("The End")
H
e
l
l
o
W
o
r
l
d
The End
Python continue
statement#
In Python, the continue
statement is a jump statement which is used to skip execution of current iteration. After skipping, loop continue
with next iteration. We can use continue
statement only with for loop as well as while loop in Python. It is typically only used within an if statement (otherwise the remainder of the loop would never be executed).
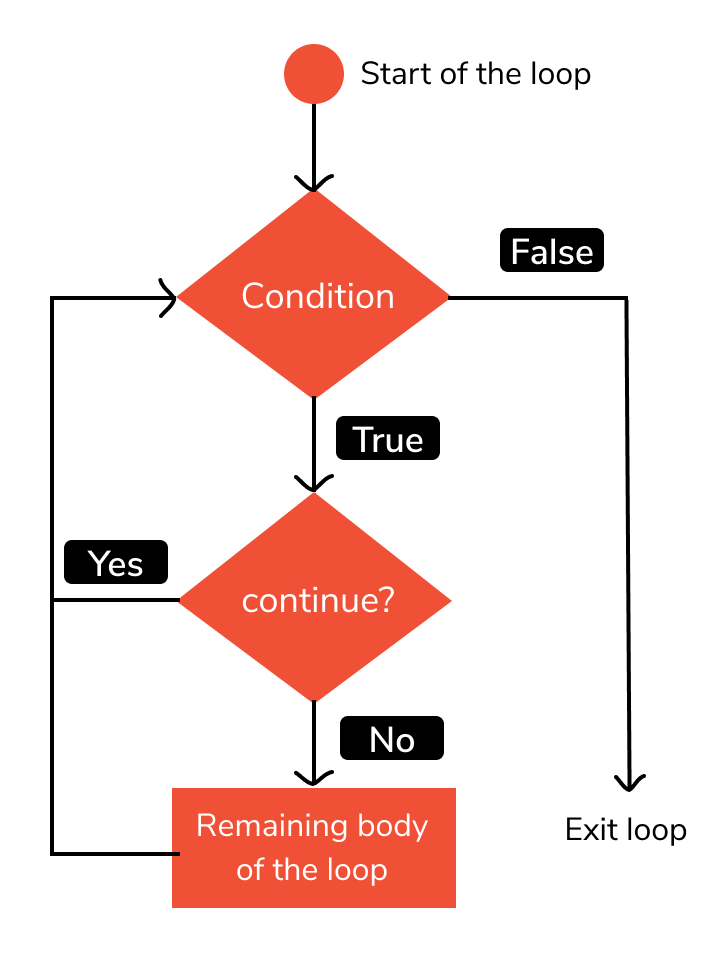
The working of continue
statement in for
loop and while
loop is shown below.
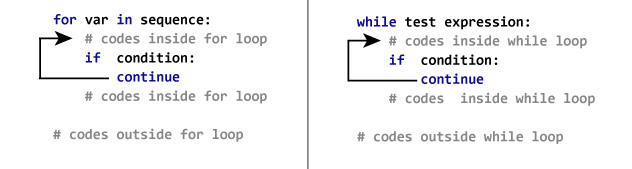
continue
statement in while
loop#
We can also use the continue
statement inside a while
loop. For example:
Write a while loop to display each character from a string and if a character is a space, then don’t display it and move to the next character.
Use the if condition with the continue
statement to jump to the next iteration. If the current character is space, then the condition evaluates to true, then the continue
statement will execute, and the loop will move to the next iteration by skipping the remeaning body.
# Example 1: use the `continue` statement inside a `while` loop
name = 'Art hu r'
size = len(name)
i = -1
# iterate loop till the last character
while i < size - 1:
i = i + 1
# skip loop body if current character is space
if name[i].isspace():
continue
# print current character
print(name[i], end=' ')
A r t h u r
continue
statement with else
statements#
Sometimes we want to know if a loop exited ‘normally’ or via a break
statement. This can be achieved with an else:
statement in a loop which only executes if there was no break
statement.
# Example 1: program to check if letter 'A' is present in the input
a = input ("Enter a word: ")
for i in a:
if(i != 'A'):
continue
else:
print("A is found")
break
print("A is not found")
# Enter a word: Honey is sweet
Enter a word: Honey is sweet
# Example 1 :without using continue
a = input ("Enter a word: ")
for i in a:
if (i == 'A'):
print("A is found")
break
else:
print ("A is not found")
# Enter a word: Honey is sweet
Enter a word: Honey is sweet
A is not found
# Example 2:
count = 0
while count < 10:
count += 1
if count % 2 == 0: # even number
count += 2
continue
elif 5 < count < 9:
break # abnormal exit if we get here!
print("count =",count)
else: # while-else
print("Normal exit with",count)
count = 1
count = 5
count = 9
Normal exit with 12
Nested continue
loops#
1. continue
Statement in Nested Loop#
To skip the current iteration of the nested loop, use the continue
statement inside the body of the inner loop. For example:
# Example 1: `continue` statement in nested loop
for i in range(1, 11):
print('Multiplication table of', i)
for j in range(1, 11):
# condition to skip current iteration
if j == 5:
continue
print(i * j, end=' ')
print('')
Multiplication table of 1
1 2 3 4 6 7 8 9 10
Multiplication table of 2
2 4 6 8 12 14 16 18 20
Multiplication table of 3
3 6 9 12 18 21 24 27 30
Multiplication table of 4
4 8 12 16 24 28 32 36 40
Multiplication table of 5
5 10 15 20 30 35 40 45 50
Multiplication table of 6
6 12 18 24 36 42 48 54 60
Multiplication table of 7
7 14 21 28 42 49 56 63 70
Multiplication table of 8
8 16 24 32 48 56 64 72 80
Multiplication table of 9
9 18 27 36 54 63 72 81 90
Multiplication table of 10
10 20 30 40 60 70 80 90 100
Explanation:
We have the outer loop and the inner loop. The outer loop iterates the first 10 numbers, and the internal loop prints the multiplication table of each number.
But if the current number of the inner loop is equal to 5, then skip the current iteration and move to the next iteration of the inner loop using the continue
statement.
2. continue
Statement in Outer Loop#
To skip the current iteration of an outside loop, use the continue
statement inside the outer loop. For example:
# Example 1: `continue` statement in outer loop
for i in range(1, 11):
# condition to skip iteration
# Don't print multiplication table of even numbers
if i % 2 == 0:
continue
print('Multiplication table of', i)
for j in range(1, 11):
print(i * j, end=' ')
print('')
Multiplication table of 1
1 2 3 4 5 6 7 8 9 10
Multiplication table of 3
3 6 9 12 15 18 21 24 27 30
Multiplication table of 5
5 10 15 20 25 30 35 40 45 50
Multiplication table of 7
7 14 21 28 35 42 49 56 63 70
Multiplication table of 9
9 18 27 36 45 54 63 72 81 90
Explanation:
The outer loop iterates the first 10 numbers, and the internal loop prints the multiplication table of each number.
But if the current number of the outer loop is even, then skip the current iteration of the outer loop and move to the next iteration.
Note: If we skip the current iteration of an outer loop, the inner loop will not be executed for that iteration because the inner loop is part of the body of an outer loop.
Catching exceptions with continue
statement#
Sometimes it is desirable to deal with errors without stopping the whole program. This can be achieved using a try statement. Appart from dealing with with system errors, it also alows aborting from somewhere deep down in nested execution. It is possible to attach multiple error handlers depending on the type of the exception
try:
code
except <Exception Type> as <variable name>:
# deal with error of this type
except:
# deal with any error
finally:
# execute irrespective of whether an exception occured or not
try:
count=0
while True:
while True:
while True:
print("Looping")
count = count + 1
if count > 3:
raise Exception("abort") # exit every loop or function
if count > 4:
raise StopIteration("I'm bored") # built in exception type
except StopIteration as e:
print("Stopped iteration:",e)
except Exception as e: # this is where we go when an exception is raised
print("Caught exception:",e)
finally:
print("All done")
Looping
Looping
Looping
Looping
Caught exception: abort
All done
This can also be useful to handle unexpected system errors more gracefully:
try:
for i in [2,1.5,0.0,3]:
inverse = 1.0/i
except Exception as e: # no matter what exception
print("Cannot calculate inverse because:", e)
Cannot calculate inverse because: float division by zero
Python pass
statement#
In Python, pass
keyword is used to execute nothing; it means, when we don’t want to execute code, the pass can be used to execute empty. It is same as the name refers to. It just makes the control to pass by without executing any code. If we want to bypass any code pass statement can be used.
It is used as a placeholder for future implementation of functions, loops, etc.
Suppose we have a loop or a function that is not implemented yet, but we want to implement it in the future. They cannot have an empty body. The interpreter would give an error. So, we use the pass
statement to construct a body that does nothing.
Example:#
'''pass is just a placeholder for
functionality to be added later.'''
>>> sequence = {'p', 'a', 's', 's'}
>>> for val in sequence:
>>> pass
We can do the same thing in an empty function or class as well.
>>> def function(args):
>>> pass
or
>>> class Example:
>>> pass
# Example 1:
months = ['January', 'June', 'March', 'April']
for mon in months:
pass
print(months)
['January', 'June', 'March', 'April']
# Example 2: Python Pass Statement
number = 0
for number in range(10):
number = number + 1
if number == 5:
pass # pass here
print('Number is ' + str(number))
print('Out of loop')
Number is 1
Number is 2
Number is 3
Number is 4
Number is 5
Number is 6
Number is 7
Number is 8
Number is 9
Number is 10
Out of loop
Explanation:
By using the pass
statement in this program, we notice that the program runs exactly as it would if there were no conditional statement in the program. The pass
statement tells the program to disregard that condition and continue to run the program as usual.
Control Statements in Python FAQs#
How many control statements are present in Python?
In Python, there are 3 types of control statements. Namely, break, continue and pass statements.
How to break from a loop in Python?
The keyword break is used to break or terminate from the current loop. The control shifts to the next statement outside the loop.
What is the difference between break and continue statements in Python?
Break statement exits out of the loop, but the continue statement shifts the control to the next iteration and doesn’t exit out of the loop.
Can we use continue in if statement in Python?
Yes. Continue statements can be used within an if statement.
What is the use of pass keyword in Python?
Pass keyword helps in avoiding errors that result due to an empty loop.